2009/06/30
Updates to Qt and Qt Creator ...
On June 25, Nokia announced the release of updates to Qt.
- Qt Creator 1.2
- Qt 4.5.2
Download is available at http://www.qtsoftware.com/downloads
2009/06/23
RFID Application
RFID Application - SQLite + Serial/Socket Programming
SQLite DB<=>logServer<=>RFID Reader(S/W)<=>RFID Reader(H/W)<=>Tag
1. Create a database for RFID Tags.
2. RFID Reader(S/W) read tag information via /dev/ttyS0.It sends the Tag ID to logServer for identification.
3. logServer receives Tag Info from RFID Reader and checks with SQLIte DB. Then feedback results to RFID Reader to take further actions. The log information is also logged into SQLite DB.
4. Log information can be browsed.
.




2009/06/21
RFID Reader - ttyS0
Refererence,
1. Serial Programming Guide for POSIX Operating Systems
2. Serial Programming HOWTO (Translated)
3. Summit RFID Reader
4. QextSerialPort ... a Qt Serial Class
Programmed as below,
#include <stdio.h> /* Standard input/output definitions */ #include <string.h> /* String function definitions */ #include <unistd.h> /* UNIX standard function definitions */ #include <fcntl.h> /* File control definitions */ #include <errno.h> /* Error number definitions */ #include <termios.h> /* POSIX terminal control definitions */ char DEV_RFID[16]="/dev/ttyS0"; int main(int argc, char **argv) { struct timeval timeout; timeout.tv_sec = 0; timeout.tv_usec = 500; // ttyS1 can be chosed if ttyS0 is in used if(argc>1) strcpy(DEV_RFID, "/dev/ttyS1"); fprintf(stdout, "[RFID] ----------- DEMO -------------------\n"); fprintf(stdout, "[RFID] Connecting to %s\n", DEV_RFID); // Open a Serial Port int fd; fd = open(DEV_RFID, O_RDWR | O_NOCTTY); if (fd < 0) { perror(DEV_RFID); exit(1); } fprintf(stdout, "[RFID] %s is connected\n", DEV_RFID); struct termios oldtio, newtio; // Gets the current serial port settings. tcgetattr(fd, &oldtio); bzero(&newtio, sizeof(newtio)); // Termios Structure Members -------------------------------------- // Control options, 19200 baud/8 data bits/Enable receiver/Local line newtio.c_cflag |= (B19200|CS8|CREAD|CLOCAL); newtio.c_lflag &= (~(ICANON|ECHO|ECHOE|ECHOK|ECHONL|ISIG)); // Input options newtio.c_iflag &= (~(INPCK|IGNPAR|PARMRK|ISTRIP|ICRNL|IXANY)); // Output options newtio.c_oflag &= (~OPOST); // Control characters newtio.c_cc[VMIN] = 0; newtio.c_cc[VTIME] = 5; // no flow control newtio.c_cflag&=(~CRTSCTS); newtio.c_iflag&=(~(IXON|IXOFF|IXANY)); // Flushes the input and/or output queue. tcflush(fd, TCIFLUSH); // Make changes now without waiting for data to complete tcsetattr(fd, TCSANOW, &newtio); // Variables for Read/Write char cmd[3] = { 0x1B, 'I', 0x0D }; unsigned char buf[256]={0} ; char id[32]={0}, chs[2]={0}; int i=0, res; while(1) { bzero(id, sizeof(id)); fprintf(stdout, "[RFID] Reading ...\n"); // Send Request to RFID Reader write(fd, cmd, 3); // Read Response from RFID Reader res = read(fd, buf, 16); // Print the Response in Hex if(res > 0) { // Convert Response into Hex Format // For checking the Respones String for(i=0; i<res; i++) { sprintf(chs, "%02X", buf[i]); strcat(id, chs ); } fprintf(stdout, "[RFID] Card ID: "); fprintf(stdout, " %s\n", id); } sleep(3); } // Close DEV_RFID used. close(fd); fprintf(stdout, "\n[RFID] Closed\n"); // Restore old terminal settings tcsetattr(fd, TCSANOW, &oldtio); fprintf(stdout, "[RFID] ---------- End of Program ----------\n"); return 0; }$ ./myRFIDreader 1 [RFID] ----------- DEMO ------------------- [RFID] Connecting to /dev/ttyS1 [RFID] /dev/ttyS1 is connected [RFID] Reading ... [RFID] Card ID: 1B054661696C21 (Nothing read return value) [RFID] Reading ... [RFID] Card ID: 1B054661696C21 [RFID] Reading ... [RFID] Card ID: 1B0E0F3871C1CD0A (Part of Card 1) [RFID] Reading ... [RFID] Card ID: 8007E000003F038B (Part of Card 1) [RFID] Reading ... [RFID] Card ID: 1B0E0F3058C1CD0A8007E000003F038B (Card 1 No.) [RFID] Reading ... [RFID] Card ID: 1B054661696C21 [RFID] Reading ... [RFID] Card ID: 1B054661696C21 [RFID] Reading ... [RFID] Card ID: 1B0E0F3871C1CD0A8007E000003F038B (Card 2 No.) [RFID] Reading ... [RFID] Card ID: 1B054661696C21 [RFID] Reading ... [RFID] Card ID: 1B054661696C21 .
2009/06/03
Bluetooth - Programming
Codes modified from Bluetooth Essential for Programmer.
#include <stdio.h> #include <unistd.h> #include <sys/socket.h> #include <bluetooth/bluetooth.h> #include <bluetooth/rfcomm.h> int main(int argc, char **argv) { struct sockaddr_rc addr = { 0 }; int s, status, bytes_read; char buf[1] = { 0 }; char dest[18] = "00:0B:0D:6C:36:0B"; // allocate a socket s = socket(AF_BLUETOOTH, SOCK_STREAM, BTPROTO_RFCOMM); // set the connection parameters (who to connect to) addr.rc_family = AF_BLUETOOTH; addr.rc_channel = 1; str2ba( dest, &addr.rc_bdaddr ); // connect to server status = connect(s, (struct sockaddr *)&addr, sizeof(addr)); // send a message if( 0 == status ) { while(1) { // read data from the client bytes_read = recv(s, buf, sizeof(buf), 0); //if( bytes_read > 0 ) { printf("%c", buf[0]); // } } } if( status < 0 ) perror("uh oh"); close(s); return 0; }# gcc rfcomm-client.c -lbluetooth # ./a.out $GPGGA,153226.985,2459.7755,N,12128.0203,E,0,00,,32.1,M,15.2,M,,0000*43 $GPRMC,153226.985,V,2459.7755,N,12128.0203,E,,,030609,,,N*7C $GPVTG,,T,,M,,N,,K,N*2C $GPGGA,153227.985,2459.7755,N,12128.0203,E,0,00,,32.1,M,15.2,M,,0000*42 $GPGSA,A,1,,,,,,,,,,,,,,,*1E $GPGSV,3,1,12,11,79,010,17,07,68,190,,08,63,334,,25,46,185,*70 $GPGSV,3,2,12,17,27,267,,28,20,334,,19,20,025,,32,10,117,*7D $GPGSV,3,3,12,22,08,029,,15,08,331,16,20,07,144,14,03,03,050,*77 $GPRMC,153227.985,V,2459.7755,N,12128.0203,E,,,030609,,,N*7D
Bluetooth - Resources
Bluez is the Official Linux Bluetooth protocol stack.
Bluetooth Essential for Programmer has some sample codes.
An Introduction to Bluetooth Programming
...
Bluetooth - GPSlim236 GPS (2)
1. Scan if any bluetooth device here ...
# hcitool scan
Scanning ...
00:0B:0D:6C:36:0B HOLUX GPSlim236
2. Bind the RFCOMM device to the remote bluetooth device.
# rfcomm bind /dev/rfcomm0 00:0B:0D:6C:36:0B
3. Check the RFCOMM device is created or not.
# ls -al /dev/rfcomm0
crw-rw---- 1 root root 216, 0 2009-06-03 21:19 /dev/rfcomm0
4. Check current RFCOMM device informaion.
# rfcomm -a
rfcomm0: 00:0B:0D:6C:36:0B channel 1 closed
5. Check if we can read data from my GPS in other terminal ...
# cat /dev/rfcomm0
$GPGGA,143236.991,2459.7755,N,12128.0203,E,0,00,,32.1,M,15.2,M,,0000*46
$GPRMC,143236.991,V,2459.7755,N,12128.0203,E,,,030609,,,N*79
$GPVTG,,T,,M,,N,,K,N*2C
$GPGGA,143237.991,2459.7755,N,12128.0203,E,0,00,,32.1,M,15.2,M,,0000*47
$GPGSA,A,1,,,,,,,,,,,,,,,*1E
$GPGSV,3,1,12,11,85,202,,07,76,233,,25,58,195,,08,48,328,17*7D
$GPGSV,3,2,12,19,31,022,,17,16,257,,28,14,321,,03,13,047,*7D
$GPGSV,3,3,12,32,07,130,,13,07,214,,22,05,042,,20,04,156,*7B
:
:
WoW...It really works :)
6. Check RFCOMM device information again.
# rfcomm -a
rfcomm0: 00:0B:0D:6C:36:0B channel 1 connected [tty-attached]
7. Remember Bluetooth Icon in system tray, select Preference Setting, a connected icon is shown.
You may stop receive data from GPS by click the disconnect button ! The cat /dev/rfcomm0 will stop then.
.
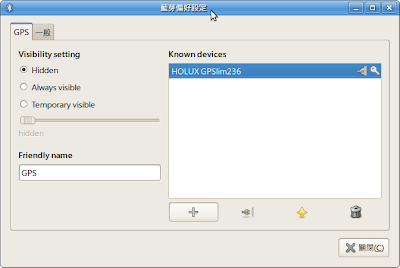
Bluetooth - GPSlim236 GPS
Bluetooth is supported in Linux kernel 2.4 and 2.6.
Holux GPSlim236 GPS information can be found in http://www.holux.com/JCore/en/products/products_content.jsp?pno=340
To make GPSlim236 works in Ubuntu 9.04,
1. Insert an USB Bluetooth Dongle.(D-Link DBT-122T)
2. The device shall be detected automatically by Ubuntu, and the bluetooth icon shall appear in the system tray.
3. Right click the bluetooth icon and select Preference Setting. Then the Preference Setting window will appear as below.
4. Give an Friendly name (GPS) and click + button to continue.
Click FORWARD button.
5. Select Use fixed PIN Code, and select pin code 0000 in my case.
Bluetooth devices detected may appear in window few seconds later.
6. Choose HOLUX GPSlim236 and Click FORWARD button.
Few seconds later, succesfully configuration message will appear.
Next we have to check if the GPS data can be read exactly.
to be continued ...
.






訂閱:
文章 (Atom)